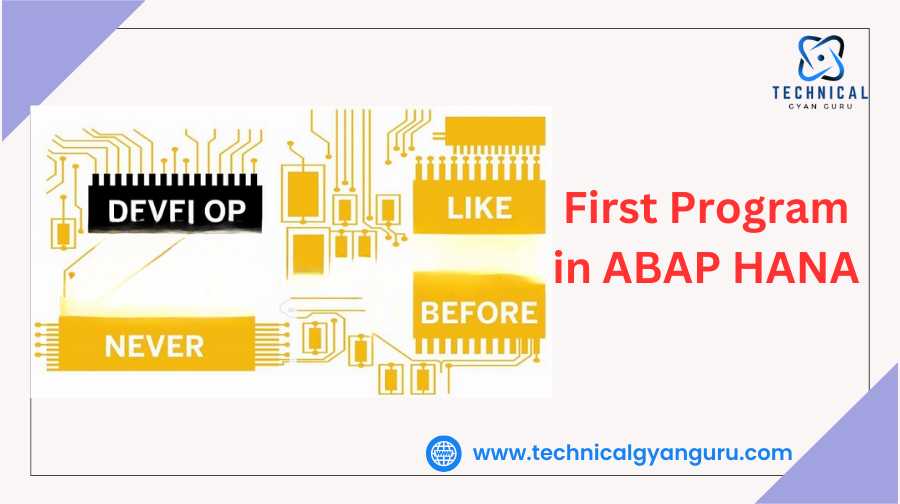
Learn how to write your first ABAP program in SAP HANA. A beginner’s guide to starting with ABAP on the HANA platform, step-by-step instructions included
ABAP (Advanced Business Application Programming) has been the cornerstone of SAP development for decades. With the advent of SAP HANA, a high-performance in-memory database, the landscape of ABAP development has transformed. This guide will walk you through creating your first program in ABAP for SAP HANA, covering all the essentials from setup to execution.
Prerequisites
Before diving into the programming aspect, ensure you have the following prerequisites in place:
- Access to an SAP HANA system
- ABAP Development Tools (ADT) installed in Eclipse
- Basic knowledge of ABAP programming
Setting Up Your Environment
- Install Eclipse: Download and install Eclipse IDE for Java Developers from the Eclipse website.
- Install ABAP Development Tools (ADT):
- Open Eclipse.
- Go to
Help
>Eclipse Marketplace
. - Search for “ABAP Development Tools” and install it.
- Restart Eclipse once the installation is complete.
- Configure the SAP System:
- Open Eclipse and go to
Window
>Perspective
>Open Perspective
>Other...
. - Select
ABAP
and clickOpen
. - In the ABAP perspective, go to
File
>New
>ABAP Project
. - Enter your SAP system connection details and click
Next
. - Enter your SAP credentials and click
Finish
.
- Open Eclipse and go to
Creating Your First ABAP Pakage
Step 1 -> Select New > ABAP Package.
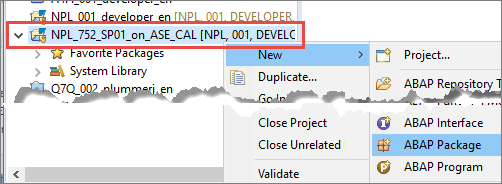
Step 2 -> Title = Zxx_TUTORIALS, where xx represents your initials.
Keywords = Table Tutorial
Packages Type = Development.
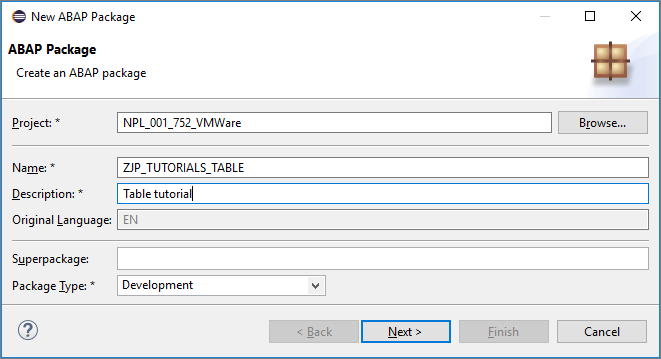
Step 3
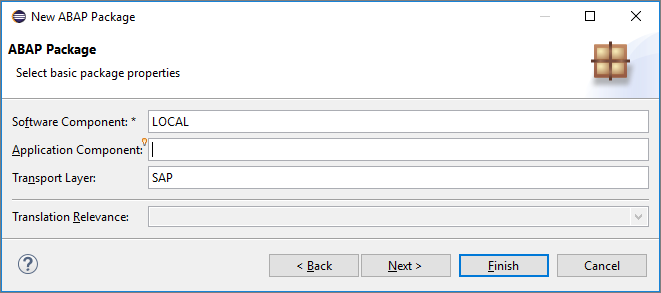
Step 4
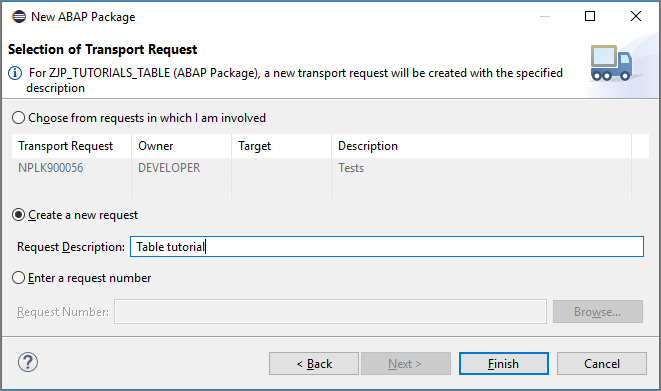
- In the Project Explorer, right-click on your ABAP project and select
New
>ABAP Package
. - Enter the package name (e.g.,
Z_FIRST_PROGRAM
) and description. - Click
Next
and thenFinish
. - Create a New ABAP Class:
- Right-click on your package and select
New
>ABAP Class
. - Enter the class name (e.g.,
ZCL_HELLO_WORLD
) and description. - Click
Next
and thenFinish
.
- Right-click on your package and select
- Write Your First ABAP Program: Open the class file you just created and enter the following code:abap
CLASS zcl_hello_world DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. METHODS: display_message. ENDCLASS. CLASS zcl_hello_world IMPLEMENTATION. METHOD display_message. WRITE: 'Hello, ABAP HANA World!'. ENDMETHOD. ENDCLASS. START-OF-SELECTION. DATA: lo_hello_world TYPE REF TO zcl_hello_world. CREATE OBJECT lo_hello_world. lo_hello_world->display_message( ).
- Activate and Run Your Program:
- Save and activate the class by clicking the
Activate
icon or pressingCtrl+F3
. - To execute the program, go to
Run
>Run As
>ABAP Application
.
- Save and activate the class by clicking the
Understanding the Code
Let’s break down the code to understand its components:
- CLASS zcl_hello_world DEFINITION: This defines a new class named
zcl_hello_world
. - METHODS: display_message: This defines a public method named
display_message
. - CLASS zcl_hello_world IMPLEMENTATION: This section contains the implementation of the methods defined in the class.
- METHOD display_message: This method contains the code to display a message.
- START-OF-SELECTION: This event block is triggered at the start of the program execution.
- DATA: lo_hello_world TYPE REF TO zcl_hello_world: This line declares a reference variable of type
zcl_hello_world
. - CREATE OBJECT lo_hello_world: This line creates an instance of the
zcl_hello_world
class. - lo_hello_world->display_message( ): This line calls the
display_message
method of thezcl_hello_world
instance.
Leveraging SAP HANA Capabilities
With SAP HANA, you can enhance your ABAP programs by leveraging its in-memory computing capabilities. Let’s extend our example to perform a simple database query using HANA.
- Create a Database Table:
- In the SAP GUI, use transaction code
SE11
to create a new database table (e.g.,ZHELLO_WORLD
). - Define the table fields (e.g.,
ID
andMESSAGE
) and activate the table.
- In the SAP GUI, use transaction code
- Insert Data into the Table:
- Use transaction code
SE38
to create a new ABAP report program (e.g.,ZINSERT_HELLO_WORLD
). - Enter the following code to insert data into the table:
REPORT zinsert_hello_world. DATA: lt_hello_world TYPE TABLE OF zhello_world, ls_hello_world TYPE zhello_world. ls_hello_world-id = 1. ls_hello_world-message = 'Hello from SAP HANA!'. APPEND ls_hello_world TO lt_hello_world. INSERT zhello_world FROM TABLE lt_hello_world.
- Use transaction code
- Extend the ABAP Class to Query the Table:
- Modify the
display_message
method to query theZHELLO_WORLD
table and display the message:
METHOD display_message. DATA: lt_hello_world TYPE TABLE OF zhello_world, ls_hello_world TYPE zhello_world. SELECT * FROM zhello_world INTO TABLE lt_hello_world. LOOP AT lt_hello_world INTO ls_hello_world. WRITE: / ls_hello_world-message. ENDLOOP. ENDMETHOD.
- Modify the
- Run the Extended Program:
- Save and activate the class.
- Execute the program again. It should now display the message stored in the
ZHELLO_WORLD
table.
Conclusion
Congratulations! You have successfully created your first program in ABAP for SAP HANA. This guide has introduced you to setting up your development environment, writing a basic ABAP program, and leveraging SAP HANA capabilities for database operations. As you continue to explore ABAP on HANA, you will discover more advanced features and optimizations to enhance your SAP applications.
you may be interested in this blog here:-
Top SAP Modules in Demand 2024 Insights & Trends
Advanced OOP Concepts in SAP ABAP A Comprehensive Guide
GRC Security: Ensuring Comprehensive Governance, Risk, and Compliance