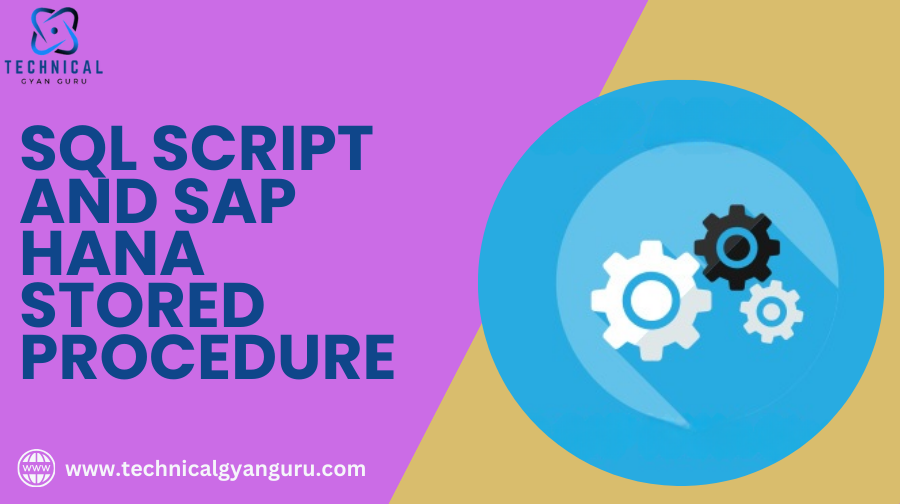
Optimize SAP HANA performance with SQL Script stored procedures. Learn to write efficient code, handle complex logic, and improve data processing speed.
In the realm of modern Database Mangment, SAP HANA stands out as a high-performance, in-memory database that provides real-time data processing capabilities. One of the key features that make SAP HANA so powerful is its support for SQL Script and stored procedures. These tools allow developers and database administrators to execute complex logic directly within the database, significantly improving performance and efficiency.
This comprehensive guide delves into the intricacies of SQL Script and SAP HANA stored procedures, exploring their syntax, usage, and best practices.
What is SQL Script?
SQL Script is an extension of SQL that provides additional capabilities for procedural logic and control-flow within SAP HANA. It allows developers to write complex queries and procedural code, which can be executed in the database engine to achieve higher performance.
Using SQL scripts
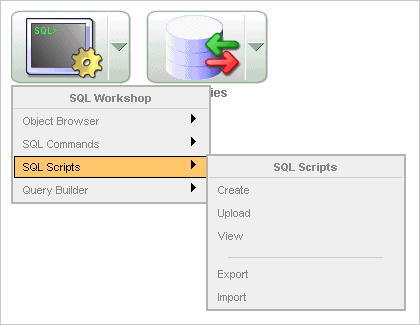
Regarding the SQL scripts page.
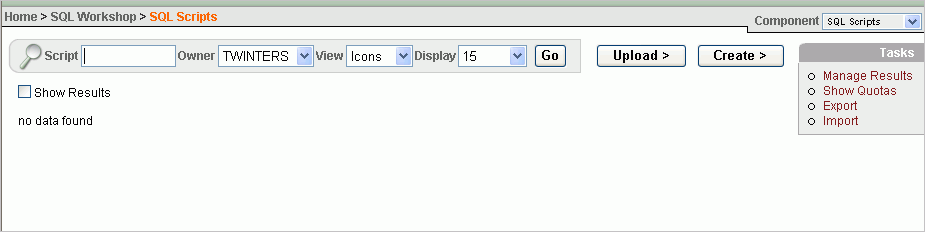
Applying the Script Editor
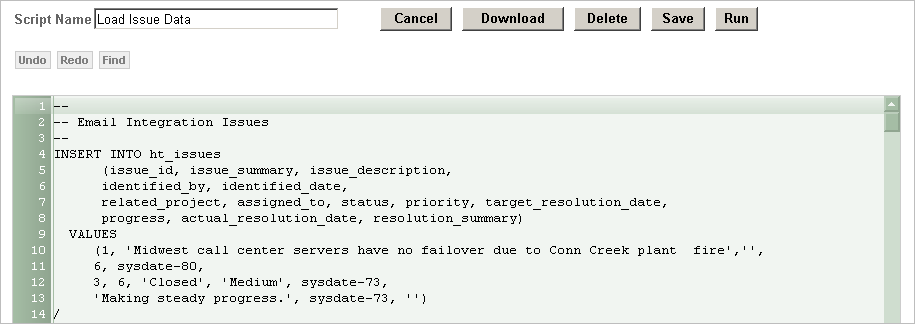
Find and Delete Text or Regular Expressions
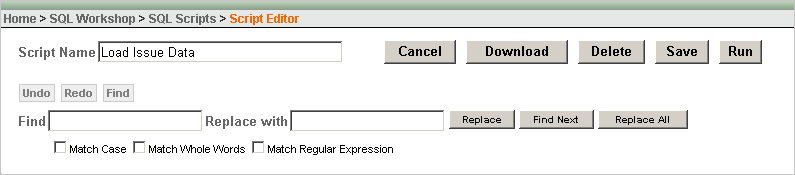
Showing Result via the SQL Scripts Page
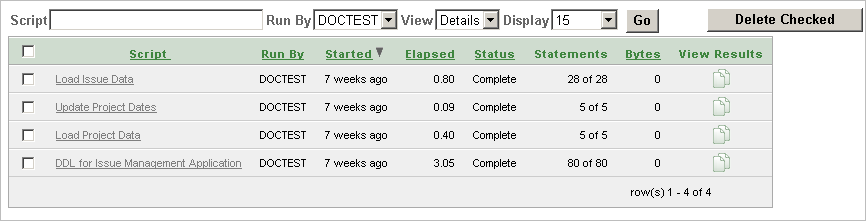
Visit the Results Page.
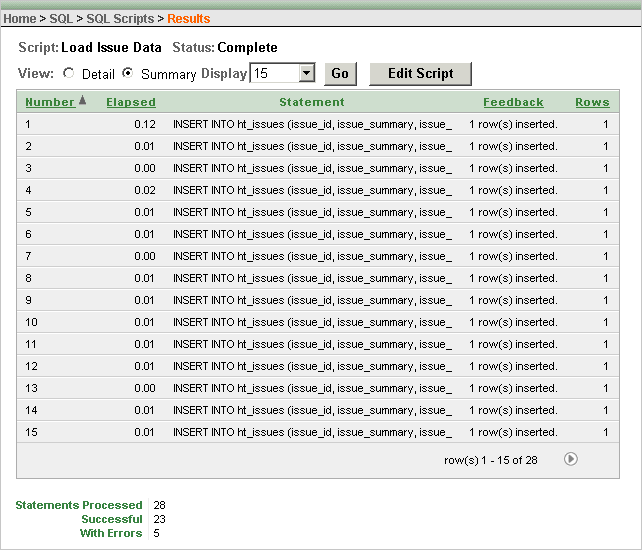
Key Features of SQL Script:
- Enhanced SQL Functionality: SQL Script extends standard SQL with additional features like loops, conditionals, and error handling.
- Increased Performance: By executing logic within the database, SQL Script reduces the need for data transfer between the application and the database, thus improving performance.
- Scalability: SQL Script supports parallel execution, making it suitable for handling large datasets and complex operations.
Understanding SAP HANA Stored Procedures
Stored procedures in SAP HANA are a set of SQL statements and procedural logic that are stored in the database and can be executed on demand. They encapsulate complex operations, making them reusable and maintainable.
Benefits of Using Stored Procedures:
- Modularity: Stored procedures promote code reuse and modularity by encapsulating frequently used logic.
- Security: They provide a secure way to execute complex logic without exposing underlying SQL code to end-users.
- Performance Optimization: By reducing network traffic and allowing for execution plan optimization, stored procedures enhance overall performance.
Getting Started with SQL Script and Stored Procedures
To harness the power of SQL Script and stored procedures in SAP HANA, it’s essential to understand their syntax and basic structure. Let’s begin with a simple example of creating a stored procedure using SQL Script.
Creating a Simple Stored Procedure:
sqlCREATE PROCEDURE my_first_procedure (IN input_param INT, OUT output_param INT)
LANGUAGE SQLSCRIPT
AS
BEGIN
DECLARE temp_var INT;
temp_var := :input_param * 2;
output_param := temp_var;
END;
In this example:
- my_first_procedure is the name of the stored procedure.
- input_param is an input parameter of type INT.
- output_param is an output parameter of type INT.
- The BEGIN…END block contains the procedural logic, which in this case, multiplies the input parameter by 2 and assigns it to the output parameter.
Advanced SQL Script Features
As you become more comfortable with SQL Script, you can leverage its advanced features to write more complex and efficient code. Here are some key advanced features:
1. Conditional Statements: Conditional statements allow you to execute different blocks of code based on certain conditions.
sqlCREATE PROCEDURE check_value (IN input_val INT, OUT result_val VARCHAR(20))
LANGUAGE SQLSCRIPT
AS
BEGIN
IF :input_val > 0 THEN
result_val := 'Positive';
ELSEIF :input_val < 0 THEN
result_val := 'Negative';
ELSE
result_val := 'Zero';
END IF;
END;
2. Looping Constructs: SQL Script supports various looping constructs such as WHILE and FOR loops.
sql CREATE PROCEDURE calculate_factorial (IN input_num INT, OUT result_num INT)
LANGUAGE SQLSCRIPT
AS
BEGIN
DECLARE i INT;
DECLARE fact INT;
fact := 1;
i := 1;
WHILE :i <= :input_num DO
fact := fact * :i;
i := :i + 1;
END WHILE;
result_num := fact;
END;
3. Error Handling: Robust error handling ensures your procedures can gracefully handle unexpected situations.
sql CREATE PROCEDURE divide_numbers (IN num1 INT, IN num2 INT, OUT result DECIMAL(10, 2))
LANGUAGE SQLSCRIPT
AS
BEGIN
DECLARE EXIT HANDLER FOR SQLEXCEPTION
BEGIN
result := NULL;
END;
IF :num2 = 0 THEN
SIGNAL SQL_EXCEPTION 'Division by zero error';
ELSE
result := :num1 / :num2;
END IF;
END;
Best Practices for Writing SQL Script and Stored Procedures
To make the most of SQL Script and stored procedures, consider the following best practices:
- Keep It Simple: Write clear and concise code. Break down complex logic into smaller, manageable procedures.
- Use Comments: Document your code with comments to improve readability and maintainability.
- Optimize for Performance: Leverage indexing, avoid unnecessary data retrieval, and use appropriate SQL constructs to optimize performance.
- Test Thoroughly: Rigorously test your stored procedures to ensure they handle all possible input scenarios and edge cases.
- Handle Exceptions Gracefully: Implement comprehensive error handling to manage exceptions and ensure your procedures fail gracefully.
Real-World Applications
SQL Script and stored procedures in SAP HANA are employed across various industries for diverse applications. Here are a few real-world scenarios where they prove invaluable:
1. Financial Analytics: Banks and financial institutions use stored procedures to perform complex calculations for risk assessment, fraud detection, and financial reporting in real-time.
2. Supply Chain Management: Manufacturing companies leverage SQL Script to optimize inventory management, track production processes, and improve supply chain efficiency.
3. Healthcare: Hospitals and healthcare providers use stored procedures to analyze patient data, manage electronic health records, and improve decision-making processes.
Conclusion
SQL Script and SAP HANA stored procedures are powerful tools that enable developers and database administrators to execute complex logic efficiently within the database. By understanding their features, syntax, and best practices, you can harness their full potential to build high-performance, scalable, and maintainable database applications.
Whether you’re optimizing financial analytics, enhancing supply chain management, or driving insights in healthcare, the knowledge and skills gained from this guide will empower you to achieve more with SAP HANA. Dive into SQL Script, experiment with stored procedures, and unlock the true potential of your data-driven applications.
Read Our blog here:-
What is the Purpose of Prompt Engineering in Gen AI Systems
Demystifying the Duolingo English Test Fee: What You Need to Know
Mastering Customer 360 in Salesforce
What is a database? Definition, Types, Uses, Advantages -2024