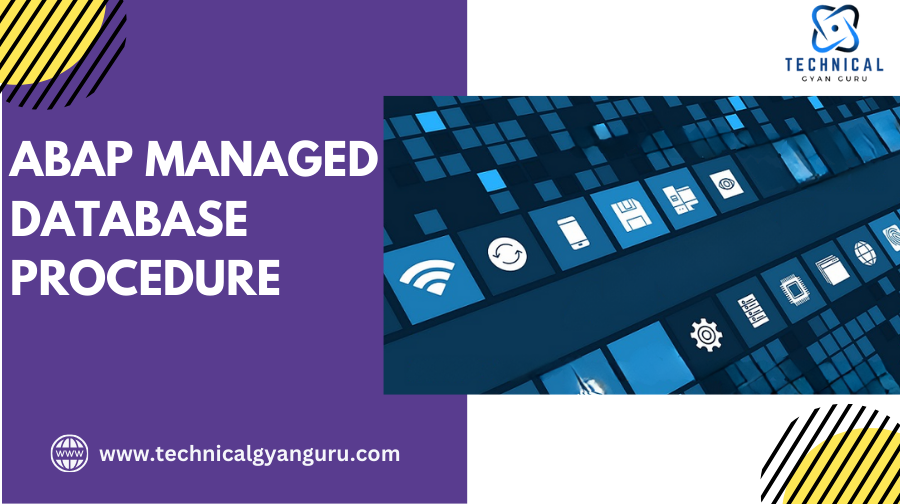
ABAP Managed Database Procedure (AMDP) lets you write database procedures directly in ABAP, enhancing performance and flexibility for complex data manipulation.
In the ever-evolving world of SAP, the need for efficient data processing and handling has led to the development of numerous advanced technologies. One such technology is the ABAP Managed Database Procedures (AMDP). AMDP represents a significant leap in the SAP ecosystem, enabling developers to leverage the power of database-specific language within ABAP programs. This article aims to provide an in-depth understanding of AMDP, its features, benefits, and practical implementation.
What is AMDP?
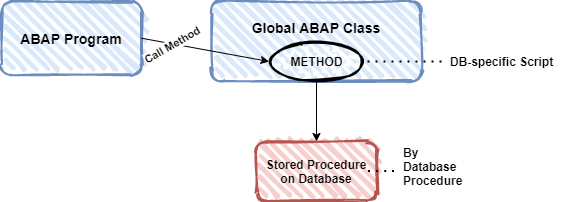
ABAP Managed Database Procedures (AMDP) is a framework that allows SAP developers to write database procedures in SQLScript, HANA SQL, and other database-specific languages directly within ABAP. These procedures are managed and executed within the ABAP environment but run natively on the database, providing performance benefits and seamless integration.

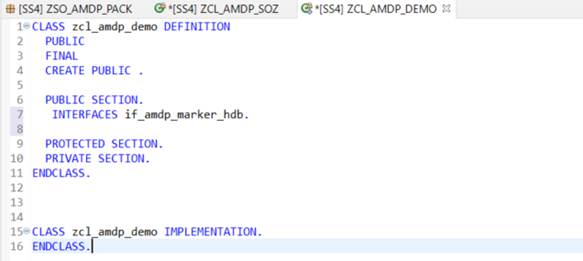
Procedure BY Database PROCEDURE|FUNCTION FOR IN .
Settings Using
Activating AMDP Debugger
CLASS zcl_amdp_demo02 DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. INTERFACES if_amdp_marker_hdb. TYPES: BEGIN OF ty_data, matnr TYPE mara-matnr, maktx TYPE makt-maktx, ersda TYPE mara-ersda, brgew TYPE mara-brgew, END OF ty_data, tt_data TYPE TABLE OF ty_data. CLASS-METHODS amdp_conversion IMPORTING VALUE(iv_data) TYPE mara-matnr EXPORTING VALUE(et_value) TYPE tt_data. PROTECTED SECTION. PRIVATE SECTION. ENDCLASS. CLASS zcl_amdp_demo02 IMPLEMENTATION. METHOD amdp_conversion BY DATABASE PROCEDURE FOR HDB LANGUAGE SQLSCRIPT OPTIONS READ-ONLY USING mara makt. et_value = select top 2 distinct mara.matnr, makt.maktx, mara.ersda, mara.brgew from mara left outer join makt on makt.matnr = mara.matnr and makt.spras = ‘E’ ; et_value2 = select mara.matnr, makt.maktx, CASE when mara.ersda >= 22102021 then ‘FUTURE’ else ‘PAST’ end as ersda, mara.brgew from mara left outer join makt on makt.matnr = mara.matnr and makt.spras = ‘E’ where mara.matnr = :iv_data ; ENDMETHOD. ENDCLASS.
Key Features of AMDP
- Seamless Integration: AMDP integrates seamlessly with the ABAP development environment, allowing developers to write and manage database procedures without leaving their familiar workspace.
- Database Agnostic: While primarily designed for SAP HANA, AMDP supports other databases, ensuring flexibility and broader applicability.
- Enhanced Performance: By executing procedures directly on the database layer, AMDP minimizes data transfer times and leverages the database’s processing power, leading to significant performance improvements.
- Code Management: AMDP procedures are version-controlled and managed within the ABAP repository, ensuring code consistency and traceability.
Benefits of Using AMDP
- Performance Optimization: AMDP procedures execute on the database server, reducing the load on the application server and optimizing overall performance.
- Simplified Development: Developers can write complex database logic within ABAP, eliminating the need for external database scripts and reducing development complexity.
- Maintainability: With AMDP, database procedures are part of the ABAP codebase, making them easier to maintain and debug.
- Flexibility: AMDP supports multiple database languages, providing flexibility in procedure development.
AMDP vs. Traditional ABAP and CDS Views
While traditional ABAP and Core Data Services (CDS) views have their place in the SAP landscape, AMDP offers distinct advantages, particularly in scenarios requiring complex data manipulation and high-performance execution.
Feature | Traditional ABAP | CDS Views | AMDP |
---|---|---|---|
Execution Layer | Application Server | Database Layer | Database Layer |
Performance | Moderate | High | Very High |
Complexity | Moderate | Low to Moderate | High (for complex logic) |
Flexibility | High | Moderate | Very High |
Use Case | General Purpose | Analytical Queries | Complex Data Processing |
Practical Implementation of AMDP
To demonstrate the practical implementation of AMDP, let’s walk through the steps of creating and using an AMDP procedure.
Step 1: Define the AMDP Class
Create an ABAP class that will contain the AMDP procedure. This class must implement the IF_AMDP_MARKER_HDB
marker interface.
abapCLASS zcl_my_amdp_class DEFINITION
PUBLIC
FINAL
CREATE PUBLIC.
PUBLIC SECTION.
INTERFACES if_amdp_marker_hdb.
CLASS-METHODS get_sales_data
IMPORTING
VALUE(iv_customer_id) TYPE kunnr
EXPORTING
VALUE(et_sales_data) TYPE TABLE OF zsales_data.
ENDCLASS.
Step 2: Implement the AMDP Method
Within the class implementation, define the AMDP method using the BY DATABASE PROCEDURE
addition.
abapCLASS zcl_my_amdp_class IMPLEMENTATION.
METHOD get_sales_data
BY DATABASE PROCEDURE
FOR HDB
LANGUAGE SQLSCRIPT
OPTIONS READ-ONLY
USING zsales.
et_sales_data = SELECT * FROM zsales WHERE customer_id = :iv_customer_id;
ENDMETHOD.
ENDCLASS.
Step 3: Calling the AMDP Procedure
Call the AMDP procedure from an ABAP program.
abapDATA: lt_sales_data TYPE TABLE OF zsales_data.
CALL METHOD zcl_my_amdp_class=>get_sales_data
EXPORTING
iv_customer_id = '000123'
IMPORTING
et_sales_data = lt_sales_data.
LOOP AT lt_sales_data INTO DATA(ls_sales_data).
WRITE: / ls_sales_data-order_id, ls_sales_data-amount.
ENDLOOP.
Advanced AMDP Features
- Exception Handling: AMDP supports exception handling, allowing developers to manage errors gracefully within the procedure.
- Table Parameters: AMDP procedures can handle table parameters, making them suitable for complex data processing tasks.
- Debugging: While debugging SQLScript directly can be challenging, AMDP provides tools to facilitate debugging within the ABAP environment.
Best Practices for AMDP Development
- Optimize SQLScript: Ensure that SQLScript within AMDP procedures is optimized for performance, avoiding unnecessary data processing.
- Use AMDP for Complex Logic: Reserve AMDP for scenarios requiring complex data manipulation that cannot be efficiently handled by CDS views or traditional ABAP.
- Monitor Performance: Regularly monitor the performance of AMDP procedures to identify and address potential bottlenecks.
- Keep It Simple: While AMDP is powerful, avoid overcomplicating procedures. Maintain simplicity and clarity in your code.
Conclusion
ABAP Managed Database Procedures (AMDP) represent a powerful tool in the SAP developer’s arsenal, combining the best of both ABAP and database-specific languages. By leveraging AMDP, developers can create high-performance, maintainable, and flexible solutions that meet the demanding requirements of modern SAP applications. Whether you’re dealing with complex data transformations or performance-critical operations, AMDP provides the capabilities needed to achieve your goals efficiently and effectively.
Read Our blog here:-
How to Reset Your KIIT SAP Portal Password Quickly
Efficient Operations and Innovative Solutions with SAP Application Management Services
How to Teach Phonices to Kids 2024 – Magic | Bright-Minds…
Cracking the Code: Your Earning Potential as a SAP ABAP Developer with 5 Years of Experience