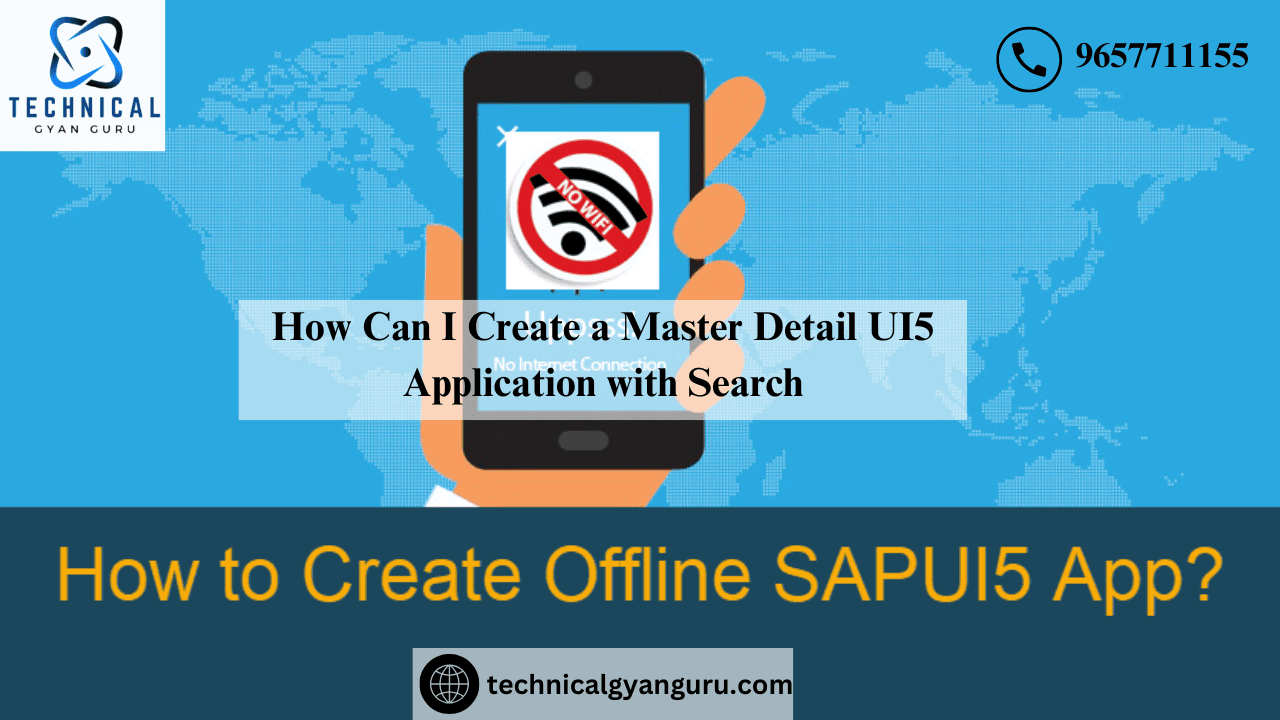
SAP offers a variety of application patterns that follow the Fiori design standards. Master Detail is one of the patterns. Split App Control can be used to accomplish it. You may find it in the sap.m library. It is intended to display a primary column on the left (1/4 of the screen size) and a secondary column on the right (3/4 of the screen size). The user can browse the list of items in the left column and choose the one that best fits their needs. The information about the selected item is shown in the wider right column.
The master view, or left column, would show a list with an Object List Item template for the items aggregate. To display the information of the clicked item on the detail page, we would use routing with a parameter when clicking the ObjectListItem. The information about the project would be displayed by another List control on the detail page.
Now let’s get going.
Create a new project from template with your SAP Web IDE. Put “demomasterdetail” on it. Your project structure will seem as follows when the project creation wizard is finished.

Include SplitApp Management
Add a SplitApp control to the View1.xml file after that.The two primary aggregations under SplitApp management are masterpages and detailpages.You can add several Master Pages and multiple Detail Pages to these aggregations.Additionally, the SplitApp control offers navigation between the detail<->detail pages and the master->master pages.To keep things simple, let’s add a master page and a detail page to our SplitApp in this lesson. We’ve added a List with an Object List Item to the Master Page. We’ve added a List with Standard List Items to the Detail Page. We’ve bound these two lists using a JSON model that was made in the view controller.
Let’s also add a header toolbar to the List Control of the Master Page. There is a search area in this toolbar that performs the search and filter functions. The code for the XML view is displayed below for your reference based on this description.
<mvc:View controllerName="org.unity.DemoMasterDetail.controller.View1" xmlns:mvc="sap.ui.core.mvc" displayBlock="true" xmlns="sap.m">
<Shell id="shell">
<App id="app">
<pages>
<Page id="page" title="{i18n>title}">
<content>
<SplitApp id="splitappid" masterButtonText="Master" masterNavigate="" afterMasterNavigate="" masterButton="" detailNavigate="">
<masterPages>
<Page id="masterpageid" title="Employee Basic Info" titleLevel="Auto" showNavButton="true" showHeader="true" showSubHeader="true"
navButtonText="" navButtonTooltip="" enableScrolling="true" icon="" navButtonTap="" navButtonPress="">
<content>
<List id="emplist" items="{/emp}" headerText="Employee Data" headerDesign="Standard" footerText="End of the List" noDataText="No Employees"
showNoData="true">
<items>
<ObjectListItem type="Navigation" title="{empname}" number="{age}" numberUnit="years" intro="{empid}" press="onObjectItemPress">
<attributes>
<ObjectAttribute title="" text="{cadre}"></ObjectAttribute>
</attributes>
<firstStatus>
<ObjectStatus title="{exp}" text="{designation}"></ObjectStatus>
</firstStatus>
<secondStatus>
<ObjectStatus title="{city}" text="{country}"></ObjectStatus>
</secondStatus>
</ObjectListItem>
</items>
<headerToolbar>
<Toolbar>
<content>
<Title text="Employees" title="Search"></Title>
<ToolbarSpacer/>
<SearchField search="onSearch" width="50%"></SearchField>
</content>
</Toolbar>
</headerToolbar>
</List>
</content>
</Page>
</masterPages>
<detailPages>
<Page id="detailpageid" title="Employee Project Information" titleLevel="Auto" showNavButton="true" showHeader="true" showSubHeader="true"
navButtonText="" navButtonTooltip="" enableScrolling="true" icon="" navButtonTap="" navButtonPress="">
<content>
<List id="projectlistid" items="{projects}">
<items>
<StandardListItem title="{projectid}" description="{projectname}"></StandardListItem>
</items>
</List>
</content>
</Page>
</detailPages>
</SplitApp>
</content>
</Page>
</pages>
</App>
</Shell>
</mvc:View>
Screenshot of the XML code from the Web IDE. Three screenshots containing the entire view’s code.



Modify the View Controller
It’s time to code the Controller for the view now. We will construct a data set including employee data in the controller’s onInit() method. This data collection will be used to build a JSON model, which we will then place in the view.Please refer to the code below for the controller’s onInit() method.
onInit: function () {
var oEmpData = {
"emp": [{
"empid": "111111",
"empname": "John Walter",
"exp": "12",
"age": "40",
"city": "Tokyo",
"country": "Japan",
"designation": "Delivery Manager",
"cadre": "Band 5",
"projects": [{
"projectid": "PGIMPL",
"projectname": "P & G SAP Implementation"
}, {
"projectid": "ARMSUPPORT",
"projectname": "Aramco Support"
}]
}, {
"empid": "222222",
"empname": "Rashid Khan",
"exp": "3",
"age": "24",
"city": "Tokyo",
"country": "Japan",
"cadre": "Band 1",
"designation": "Software Engineer",
"projects": [{
"projectid": "AIRBNB",
"projectname": "AIRBNB Implementation"
}, {
"projectid": "TATA",
"projectname": "Tata Power Support"
}]
}, {
"empid": "333333",
"empname": "Supriya Singh",
"exp": "5",
"age": "34",
"city": "Tokyo",
"country": "Japan",
"cadre": "Band 3",
"designation": "Sr. Software Engineer",
"projects": [{
"projectid": "INDIAGOV",
"projectname": "INDIA GOV SAP Implementation"
}, {
"projectid": "ARMSUPPORT",
"projectname": "Aramco Support"
}]
}]
};
var oModel = new JSONModel(oEmpData);
this.getView().setModel(oModel);
},
Organize the Events
Let’s also add the code for the event handler of the onPress event of the Object List Items, which are displayed on the SplitApp’s Master page.The binding path of the item that the user touched will be determined in the onPress event handler, and we will utilize this binding path to do the element binding of the list that needs to be presented on the SplitApp Detail Page.
Please see the code attached.
onObjectItemPress: function (oEvent) {
var oItem = oEvent.getSource();
var oCtx = oItem.getBindingContext();
var path = oCtx.getPath();
this.getView().byId("projectlistid").bindElement(path);
},
Ability to Search
Let’s go ahead and write the code for an additional event handler for the event that is generated when a user searches the Master List. The code is shown below. Every line of this code is explained in the comments put within it.
onSearch: function (oEvent) {
// create a blank filter array
var aFilter = [];
// get the string which was searched by the user
var sQuery = oEvent.getParameter("query");
// create new filter object using the searched string
var oFilter = new sap.ui.model.Filter("empname", FilterOperator.Contains, sQuery);
// push the newly created filter object in the blank filter array created above.
aFilter.push(oFilter);
// get the binding of items aggregation of the List
var oBinding = this.getView().byId("emplist").getBinding("items");
// apply filter on the obtained binding
oBinding.filter(aFilter);
}
During the onSearch event, we are carrying out the following tasks:
- Making a table or filter array that is empty
- obtaining the search string input
- Make use of the searched string to create the filter object.
- Transfer the filter object to the array of filters.
- Retrieve the list’s aggregation’s binding of items.
- Apply the filter last.
Requirements for Filter Function
We must define both the Filter and FilterOperator objects in the sap.ui.define declaration above the controller in order for them to function.

Testing Time
Now that the program is running, let’s check how our Master Detail program functions. Select the “run” icon that appears on your WebIDE’s toolbar. This will open a new browser window with the application. As of right now, the application is not available on any repository, including ABAP, Cloud, GIT, etc. The SAP WebIDE is the operating system.
Final Product…
Since no employee has been chosen by default, the detail page displays a message that reads “No Data” instead of any project information.

By virtue of element binding, related project details are displayed in the Detail section when an employee’s information from the Master list is clicked.

In the Master Section’s Search Field, type a search term. The JSON Model’s empname attribute will be used to filter the list.

Entire text
What are the lessons we took away today?
- Applying SplitApp Control
- Application of the Details and Master Pages
- Linking of Elements
- Applying a Filter to List Management
Kindly provide feedback.
you may be interested in this blog here:-
How can I see the values of variables in OpenSolver as they change?
Navigating SAP’s E070 Table in SAP: The Heartbeat of Transport Management