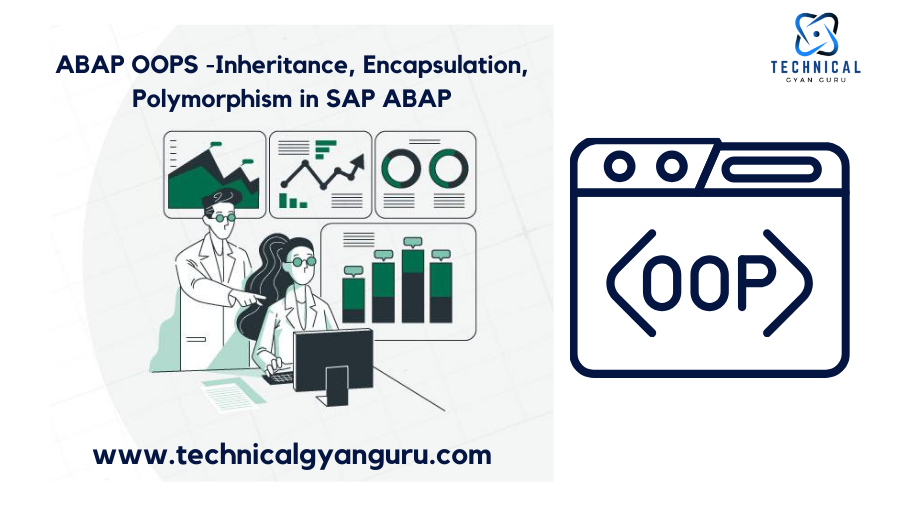
Unleash the power of reusability, data protection, and flexible code in Polymorphism in SAP ABAP! Dive deep into OOP concepts like Inheritance, Encapsulation, and Polymorphism. Learn how to leverage these features to write cleaner, more maintainable ABAP code…
Frustrated with clunky, hard-to-maintain ABAP code? Are you tired of copy-pasting similar logic into different parts of your program? What if there was a way to write cleaner, more maintainable code that could be easily reused across your projects?
Welcome to the wonderful world of Object-Oriented Programming (OOP) in ABAP! This powerful approach to development goes beyond traditional procedural coding, offering a toolbox of features like Inheritance, Encapsulation, and Polymorphism that can transform your ABAP experience. In this comprehensive guide, we’ll delve into each of these concepts, showing you how to harness their power to write more efficient, flexible, and future-proof ABAP code.
Polymorphism in SAP ABAP
Inheritance is a cornerstone of object-oriented programming (OOP) that allows you to establish hierarchical relationships between classes in ABAP. Imagine a complex family tree, where each generation inherits certain traits from the previous one. In ABAP inheritance, a new class, called the subclass, inherits the attributes and methods of an existing class, known as the superclass. This powerful concept promotes code reusability, reduces development time, and fosters a more organized code structure.
Reusing Code Through Inheritance
One of the most significant advantages of inheritance is the ability to reuse existing code. When you define a superclass, you essentially create a blueprint that captures the common functionalities shared by a group of related objects. Subclasses then inherit these functionalities, eliminating the need to rewrite them for each individual class.
Let’s illustrate this with a practical example. Imagine you’re building an Polymorphism in SAP ABAP program to manage different types of vehicles. You could create a base class named “Vehicle” that defines attributes like model
, color
, and engine_type
. It could also contain methods like display_vehicle_info()
to showcase this information. Now, you can create subclasses like “Car” and “Truck” that inherit everything from “Vehicle”. These subclasses can then add their own specific attributes and methods, such as “number_of_doors” for “Car” or “cargo_capacity” for “Truck”. This approach saves you time and effort while ensuring consistency in your codebase.
In essence, inheritance allows you to leverage the common functionalities defined in the superclass while specializing each subclass with its unique characteristics. This promotes code efficiency and reduces redundancy.
Public vs. Private vs. Protected Access Specifiers in Inheritance
Inheritance in ABAP interacts seamlessly with access specifiers, which control the visibility and accessibility of class attributes and methods. Understanding these specifiers is crucial for effective inheritance.
- Public: Members declared as public in the superclass are freely accessible from both the superclass itself and any subclasses that inherit from it. This allows for direct usage of these elements within the subclasses.
- Private: Private members are encapsulated within the superclass and are not directly accessible from subclasses. However, subclasses can still indirectly access private members through public getter methods defined in the superclass. This enforces data protection and promotes controlled access.
- Protected: Protected members offer a middle ground. They are not directly accessible from outside the class hierarchy but can be accessed from the superclass itself and all its subclasses. This allows subclasses to utilize these members without granting access to external code.
Choosing the appropriate access specifier for each member in your superclass is essential for maintaining data integrity and promoting a well-structured inheritance hierarchy. By effectively combining inheritance with access specifiers, you can achieve a balance between code reusability and data protection within your ABAP applications.
Encapsulation in ABAP
Encapsulation is a fundamental principle of Object-Oriented Programming (OOP) that binds together data (attributes) and the functions (methods) that manipulate that data, and keeps both safe from outside interference and misuse. In ABAP, encapsulation allows you to create well-defined and secure objects that manage their own internal state and behavior. Let’s delve deeper into how encapsulation works in ABAP and the benefits it brings to your code.
Binding Data and Methods within a Class
The core idea behind encapsulation is to create a protective barrier around an object’s internal details. This includes both the data it holds (attributes) and the functions that operate on that data (methods). By grouping these elements within a class definition, you establish a clear boundary between the object’s internal workings and how it interacts with the outside world.
ABAP classes provide three access specifiers to control how attributes and methods are accessed:
- Public: Public members are accessible from anywhere in your ABAP program. This allows for easy interaction with the object’s functionality.
- Protected: Protected members are accessible only within the class itself and by its subclasses (through inheritance). This ensures that core functionalities remain accessible for customization while maintaining some level of control.
- Private: Private members are strictly hidden within the class and cannot be accessed directly from outside. They can only be accessed and modified by the class’s own methods. This provides the highest level of data protection and enforces proper data manipulation through designated methods.
By strategically using these access specifiers, you can ensure that an object’s critical data is shielded from accidental modification while still allowing for controlled interaction through its public methods. This not only improves data integrity but also promotes modularity and reusability of your code.
Protecting Data Integrity through Access Specifiers
Let’s take a practical example to illustrate how encapsulation safeguards data integrity. Imagine you’re building an ABAP class to represent a “Customer” object. This class would likely have attributes like customer ID, name, and address. Encapsulation allows you to:
- Declare these attributes as private: This prevents any external code from directly modifying the customer data. For instance, another program couldn’t accidentally overwrite a customer’s name.
- Provide public methods for accessing and modifying data: You can create methods like
get_customer_name()
andupdate_customer_address()
. These methods would handle the internal logic of retrieving or updating the data while ensuring that any validation or formatting rules are applied before modifying the private attributes.
This approach ensures that all data manipulation happens through controlled channels, minimizing the risk of errors and maintaining data consistency. Additionally, you can modify the internal behavior of these methods (like adding new validation checks) without affecting how external code interacts with the “Customer” class. This promotes loose coupling and simplifies future maintenance.
Polymorphism in SAP ABAP: The Art of Flexible Code
Polymorphism, the third pillar of OOP alongside inheritance and encapsulation, adds another layer of power and flexibility to your ABAP code. It allows you to define a method with the same name in different classes, yet have the specific implementation vary depending on the object type at runtime. This enables you to write generic code that can work seamlessly with various objects within an inheritance hierarchy.
Method Overriding: Tailoring Inherited Behavior
One key aspect of polymorphism in Polymorphism in SAP ABAP is method overriding. When a subclass inherits a method from its superclass, it has the ability to redefine that method’s behavior to fit the specific needs of the subclass. This is particularly useful when dealing with shared functionality across related classes.
Imagine a base class “Vehicle” with a method “display()”. This method might simply print a generic message like “This is a vehicle”. Now, consider subclasses like “Car” and “Truck”. Overriding the “display()” method in these subclasses allows you to provide more specific information. The “Car” class might override “display()” to print “This is a Car with model XYZ”, while the “Truck” class could override it to print “This is a Truck with payload capacity of 10 tons”.
Here’s the beauty of polymorphism: When you call the “display()” method on an object reference of the base class “Vehicle”, the actual method executed will depend on the object’s type at runtime. If the object is a “Car”, the overridden “display()” specific to “Car” will be called, providing the detailed car information. This allows you to write generic code that iterates through a collection of “Vehicle” objects, calling “display()” on each one, yet automatically getting the customized output based on the actual object type.
Dynamic Binding and Late Binding: Unveiling the Magic Behind the Scenes
Polymorphism relies on two key concepts under the hood: dynamic binding and late binding. Let’s break down what these terms mean:
- Dynamic Binding: During runtime, the specific method to be executed is determined based on the actual object type.
Conclusion
In conclusion, by embracing OOP concepts like Inheritance, Encapsulation, and Polymorphism, you can unlock a new level of efficiency and maintainability in your ABAP development. Inheritance promotes code reuse through a hierarchical structure, while Encapsulation safeguards data integrity and enforces a clean separation of concerns. Polymorphism, on the other hand, injects flexibility into your code, allowing objects to respond differently to the same message.
Start leveraging these powerful tools today to streamline your ABAP development process. Explore the SAP ABAP Development Workbench, specifically Class Builder (SE21) and Object Navigator (SE80), to experiment with creating and manipulating classes. Remember, a well-designed object-oriented approach can significantly reduce development time, improve code readability, and ensure a robust foundation for future modifications. By adopting OOP principles, you’ll not only elevate the quality of your ABAP code but also become a more valuable asset in the SAP development landscape.
you may be interested in this blog here:-