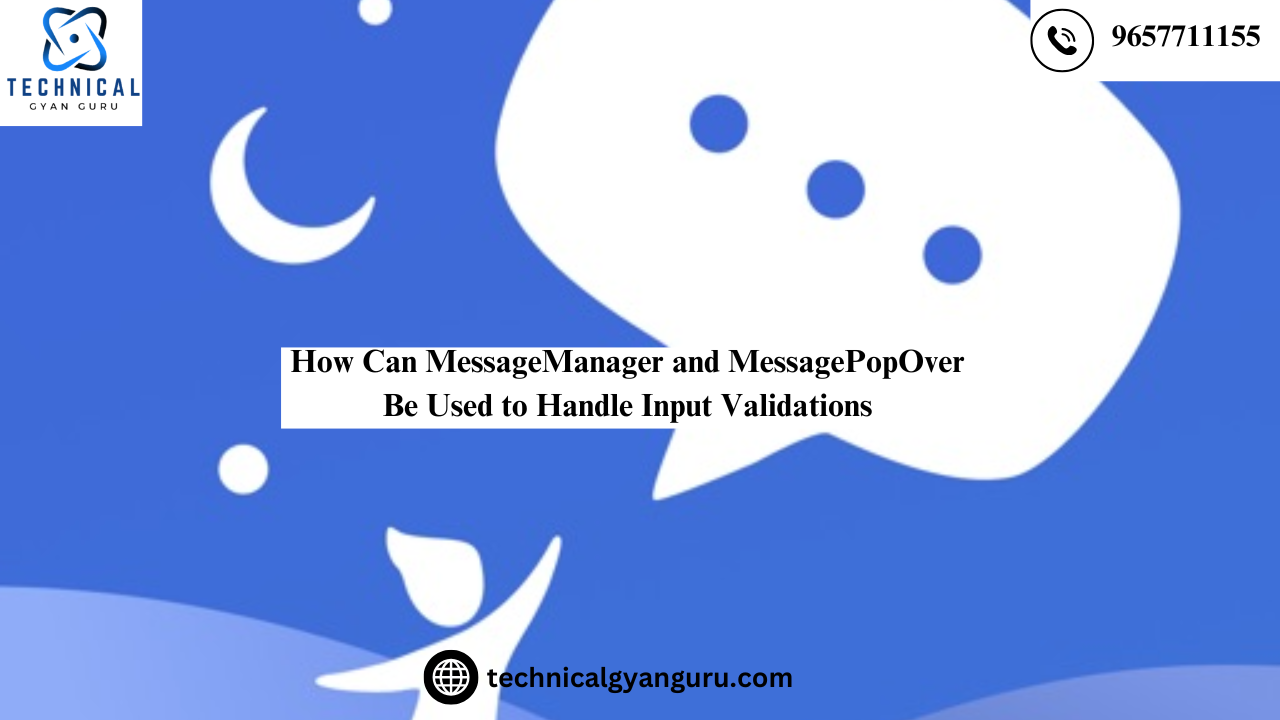
There are several approaches to writing code for input-field validations and handling error messages. Typically, we utilize pre-defined messages to assist us design different routines to handle those issues and then show those error messages to the user. When there are a lot of input fields on the screen, these duties get exceedingly tedious.
However, what if I told you that all of those error messages don’t need to be explicitly defined? What if there was a method to have all of those error messages displayed in one location as soon as the user typed anything? Indeed, it is feasible, thanks to UI5‘s feature-rich controls. View the pictures below to see what we hope to accomplish at the conclusion .


The Tutorial’s Goal
- So let’s set our goals and work toward them one at a time:
- Create the XML view by adding the MessagePopover button and input fields.
- Recognizing different input-field limitations
- Recognizing MessageManger and incorporating the view as an item.
- Adding MessagePopover to the button that appears when a message pops over.
The XML View:


With the aid of Controls like Datepicker and Input, we have constructed several input fields in the XML view. Each of these controls has its properties preserved in a JSON model:
var viewModel = new JSONModel(); var adata = { "productid": "", "pidplaceholder": "Enter Product Id", "productdesc": "", "pdesc_placeholder": "Enter Product Description", "qty_placeholder": "Enter Quantity", "quantity": "", "date": "", "vstate": "None", "vtext": "" }; viewModel.setData(adata); this.getView().setModel(viewModel, "viewinputs");
At the start of the controller, I have already imported the library “sap/ui/model/json/JSONModel.”
Recognizing input-field constraints:
We have used the “sap.ui.model.type.String” type for the product-id, and we are passing a regular expression, CO-[0-9], in the constraints to make sure the user inputs a product-id that begins with the specified regular expression. Verifying which restrictions are supported by which class is crucial. For example, “sap.ui.model.type.Integer” is not compatible with Regex, so it cannot be used there.
For the Quantity, we have used “sap.ui.model.type.Float”. The constraints contain the minimum and maximum values that can be entered into the quantity field.
We are utilizing Datepicker control to determine the delivery date. We’ve used “sap.ui.model.type.Date” for this.It is crucial to give the pattern in the formatOptions before supplying restrictions. An error will be raised if the user specifies a date that is less than this one. You can see all of these codes in the XML view snippets.
Knowing How to Use MessageManager
The various input-field types have been specified. Although these type-classes manage the data on the backend, there is currently no way to display those problems to the user interface. All errors generated for the input fields and intern are captured by the Message Manager once our view is registered with it. These errors are then sent back to the view and the relevant control. We have developed a unique message model to capture each of those messages.This is the controller’s whole code:
sap.ui.define([ "fiori/controller/BaseController", "sap/ui/model/json/JSONModel", "sap/m/MessagePopover", "sap/m/MessagePopoverItem", "fiori/model/formatter", "sap/ui/model/Filter", "sap/ui/model/FilterOperator" ], function (BaseController, JSONModel, MessagePopover, MessagePopoverItem, formatter, Filter, FilterOperator) { "use strict"; return BaseController.extend("fiori.controller.MainScreen", { formatter: formatter, onInit: function () { // create a message manager and register the message model this._oMessageManager = sap.ui.getCore().getMessageManager(); this._oMessageManager.registerObject(this.getView(), true); this.setModel(this._oMessageManager.getMessageModel(), "message"); this.oMessageTemplate = new MessagePopoverItem({ type: "{message>type}", title: "{message>message}", subtitle: "{message>additionalText}", description: "{message>description}" }); this.oMessagePopover = new MessagePopover({ items: { path: "message>/", template: this.oMessageTemplate } }); this.byId("popover").addDependent(this.oMessagePopover); var viewModel = new JSONModel(); var adata = { "productid": "", "pidplaceholder": "Enter Product Id", "productdesc": "", "pdesc_placeholder": "Enter Product Description", "qty_placeholder": "Enter Quantity", "quantity": "", "date": "", "vstate": "None", "vtext": "" }; viewModel.setData(adata); this.getView().setModel(viewModel, "viewinputs"); }, handleNext: function () { this.getRouter().navTo("DetailScreen"); }, handleSave: function (oEvent) { this.oMessagePopover.openBy(oEvent.getSource()); }, handleClear: function () { } }); });
Examine the onInit() function’s commencement. We are capturing every message in the message-model and registering the view with the message management.
Making use of MessagePopover to describe errors:
The Message Manager class is used in conjunction with Message Popover Control. Without disturbing the user, it is used to gather all error messages and compile them into a list.
The amount of errors on the Message Pop Over button must first be displayed. We wrote this code in the View with this goal in mind.

With the help of Expression-binding we found out the length of the message-model, which we declared at the time of registering the view with the message manager. It will result like this:

The Library “sap/m/MessagePopoverItem” is used to fill the Message Popover. To fill in the data in the message popover, we offer the message-model in this object. Use the addDependent() function to add the MessagePopover as a dependency of the Popover button.
this.oMessageTemplate = new MessagePopoverItem({ type: "{message>type}", title: "{message>message}", subtitle: "{message>additionalText}", description: "{message>description}" }); this.oMessagePopover = new MessagePopover({ items: { path: "message>/", template: this.oMessageTemplate } }); this.byId("popover").addDependent(this.oMessagePopover);
We are now prepared to gather the message popover errors. However, this popover must open each time the user hits the Popover button. We employ MessagePopover’s openBy() function for this. The parent object instance that is invoking the MessagePopover must be sent to this method. Since the Popover Button itself is involved in our situation, we manage it as follows:

That’s all, and we can now test the app. Recall that mistake texts are not a concern at this time.

The message manager is now handling all of these types of issues.Try it out and create your own clever validations.
This is how you should be able to gather and manage the errors. Have fun coding and designing.

Read Our blog here:-
Learn how to update function modules in SAP ABAP easily
Landing Your Dream Job: The Ultimate Guide to SAP MM Consultant resume 3 years experience