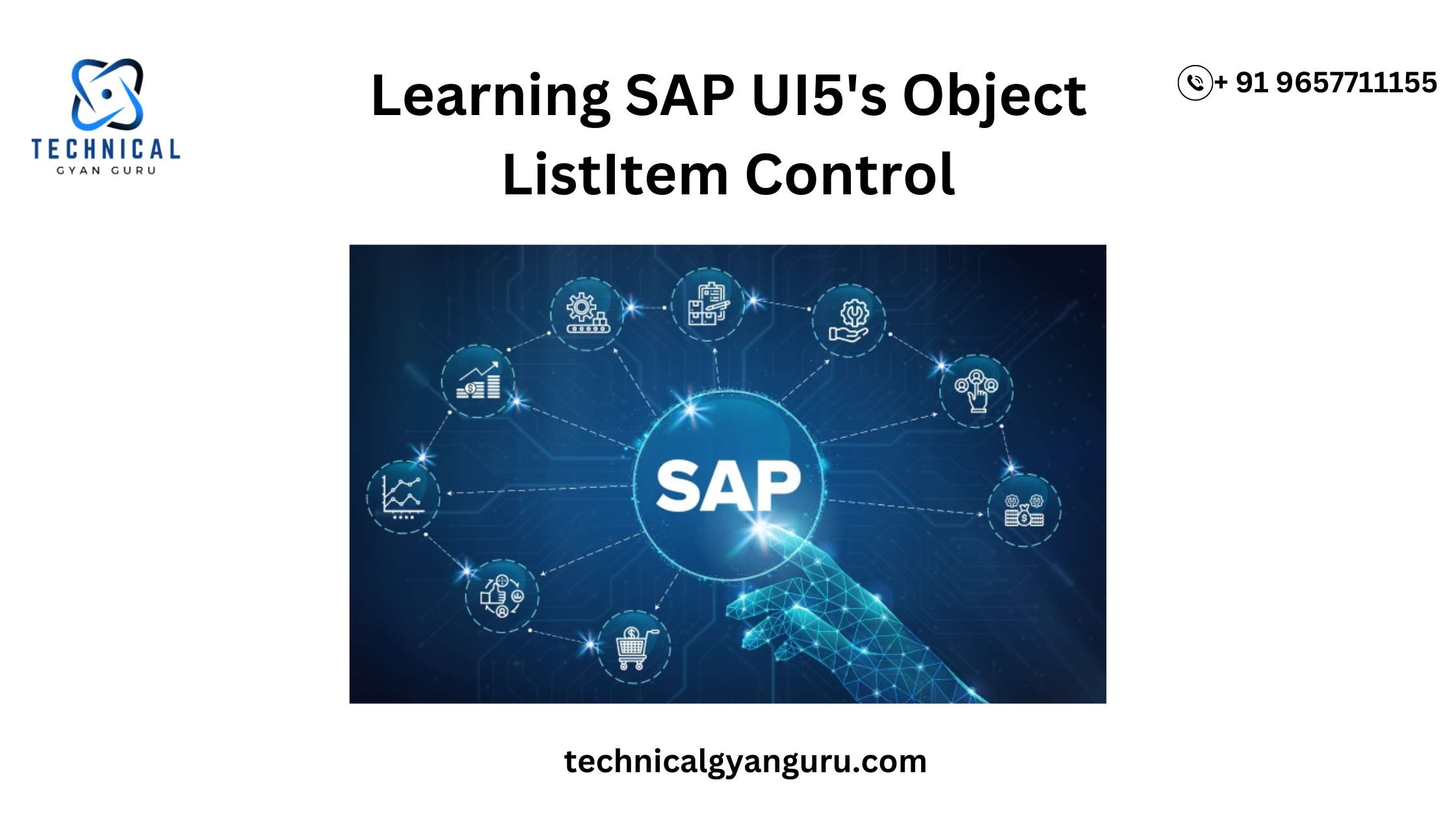
One variation of List Items in SAPUI5 is called Object List Item. A list item may be an action list, display list, feed list, standard list, or object list item. Many of us may be utilizing any or all of these list items without realizing what they mean. I’ll try to explain how to use the object list item in this lesson. SAP UI5 Object ListItem Control
Let’s improve the same code such that an Object ListItem is used in place of the StandardListItem.
Here is the earlier piece I mentioned:
SAPUI5: Using the SAP Web IDE to Bind JSON Model Data in SAPUI5 List Control
An ObjectListItem can furthermore display the title, key figure, attributes, and status of any item, but a StandardListItem can only display the title and description of any given object. When creating an application of the Master Detail type, such a list item is recommended. The master view of a master-detail application has a List of ObjectListItems that show an object’s most crucial characteristics. The user can choose whether or not to choose an item to examine its details depending on these attributes.
First, let’s improve our JSON model to add data that can be shown on an objectListItem. Additionally, the model needs to be improved to include the data that should be shown at the ObjectListItem press event. Thus far, we have just shown the employee’s name and ID in our employee data example. Let’s add some more parameters like staff experience (in years), location, and cadre (like band 1, band 2, etc.). The ObjectListItem would display these extra characteristics. Let’s include a list of every project the worker has contributed to. Therefore, a list of the projects that an employee has worked on should appear in another list upon clicking an ObjectListItem.
After enhancing the JSON model as described above, it will look like shown below.
onInit: function () {
var oEmpData =
{
"emp": [{
"empid": "111111",
"empname": "John Walter",
"exp": "120 months",
"age": "34",
"city": "Colombo",
"country":"Sri Lanka",
"cadre": "Band 5",
"designation": "Delivery Manager"
"projects": [{
"projectid": "PGIMPL",
"projectname": "P & G SAP Implementation"
},
{
"projectid": "ARMSUPPORT",
"projectname": "Aramco Support"
}
]
},
{
"empid": "222222",
"empname": "Rashid Khan",
"exp": "40 months",
"age": "34",
"city": "Mumbai",
"country":"India",
"cadre": "Band 1",
"designation": "Software Engineer",
"projects": [{
"projectid": "TATAIMPL",
"projectname": "Tata SAP Implementation"
},
{
"projectid": "AIRIMPL",
"projectname": "AIRBUS Implementation"
}
]
},
{
"empid": "333333",
"empname": "Supriya Singh",
"exp": "60 months",
"age": "34",
"city": "Tokyo",
"country":"Japan",
"cadre": "Band 3",
"designation": "Sr. Software Engineer",
"projects": [{
"projectid": "PGIMPL",
"projectname": "P & G SAP Implementation"
},
{
"projectid": "ARMSUPPORT",
"projectname": "Aramco Support"
}
]
}
]
};
var oModel = new JSONModel(oEmpData);
this.getView().setModel(oModel);
},
We have included the employee’s experience, age, city, country, cadre, designation, and project information in the updated JSON model.
As previously said, there are three things we are performing with the code above:
- Initially, create employee data in JSON format. Node ’emp’ is the root.
- Second, we are leveraging employee data to create an instance of the JSON model. Var oModel is equal to new JSONModel(oEmpData);
- Finally, we are assigning the model to the view so that the view’s controls can bind to its data.this.getView().setModel (model);
Let’s now add the ObjectListItem control to the view. Below is the modified View1.xml code. In the prior exercise, StandardListItem was utilized.


An additional list showing the projects the worker has completed
- An ObjectListItem Control has been added to the revised view code. Its title, number, number of units, and intro characteristics have all been utilized. Additionally, we made use of its first status and second-status aggregations.
- We have bound JSON model data to it using the aforementioned properties and aggregations. Please carefully examine the aforementioned graphics to determine which ObjectListItem property is related to which Model attribute.
- By using the type= “Navigation” parameter, we have enabled clickability for the list.
- Additionally, we have an event handler defined in the view controller named onObjectItemPress. Look over the event handler code below.
- Additionally, we have the project details displayed using StandardListItem.
onObjectItemPress:function(oEvent)
{
var oItem = oEvent.getSource();
var oCtx = oItem.getBindingContext();
var path = oCtx.getPath();
this.getView().byId("projectlistid").bindElement(path);
}
- Based on the o Event parameter, this function determines the binding path of the clicked ObjectListItem.
- After that, it handles another List control that is present in the view and makes use of Element Binding to show the data that is accessible on the path of the selected item. In our instance, it will show the employee’s project details.
This concludes it. Now let’s launch the software.

The employee’s data is shown in the output above using ObjectListItem based on binding that was completed in the XML view. Because we have the type property set to navigation, the list can be clicked. The project details for the selected employee are shown in a second list that appears beneath the first.
The code snippets in this lesson can be used to practice this activity. If you encounter any difficulty doing this, kindly remark. I will answer any questions or comments you have right away.
YOU MAY BE INTERESTED
10 Real-World SAP ABAP Programming Examples (with Code!)
Your Definitive Guide to Becoming a SAP ABAP Developer