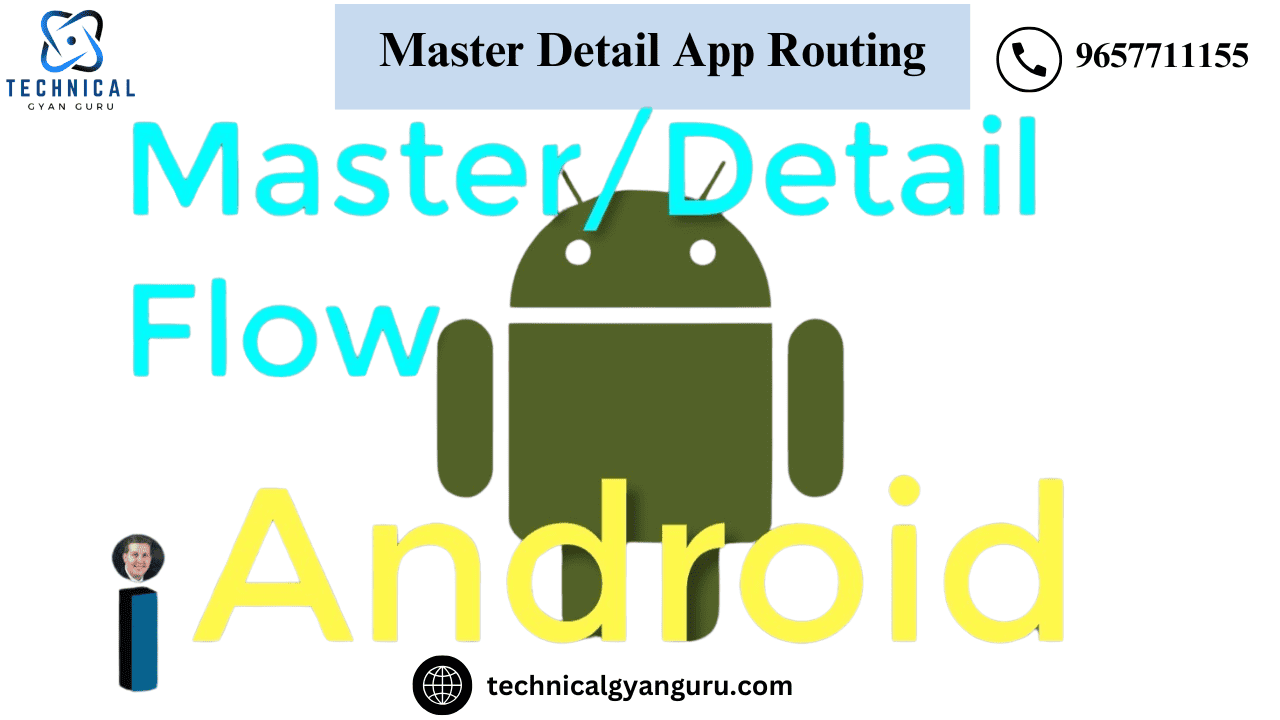
When an application contains two or more views, routing is necessary. Routing becomes simpler if the application has two or more full page views. All you have to do is include every view in an App control and use the control’s “to” method to navigate to the desired view. However, because the Master and Detail views are on the same page but belong to two separate aggregations of the Split App control, navigation in a Master Detail scenario is a little more complicated. The detail Pages aggregation is home to Detail View, while the master Pages aggregation is home to Master View. The routing setup has been completed with this in mind.
Establishing the Necessary Views
In order to accomplish navigation in a Master Detail scenario, three views are required.
- DivideAppView
- MasterView
- View in Detail
The root view, SplitAppView, will be housed inside an App Control. The SplitApp Control will be present in it.
The SplitApp Control masterPages aggregate will contain the MasterView, which is the Master view.
DetailView will exist as Detail view and be a part of the SplitApp Control detailPages aggregation.

Routing Configuration
The three words above indicate how our routing configuration will be set up.


Note: The SplitAppView and MasterView routes’ patterns are blank in the screenshot above.It indicates that when a blank pattern is found in the URL, they will be presented one after the other.SplitAppView will be rendered first because it is set up as the application’s root view (in the manifest.js file). After that, the router will be set up. Following router initialization, the URL pattern is examined, and MasterView is rendered if a blank pattern is discovered.
Furthermore, observe that the DetailVIew pattern in the setup above is product/{path}.This indicates that the pattern’s name is “product,” and the dynamic parameter “path” is what we are sending to the target during navigation.This tutorial also explains how to pass this parameter.
Every route that was previously set up has a target assigned to it. The only thing that a target is is the view that will be rendered for a specific route. The definition of targets is shown below.

For this target, four settings have been defined.
- View Name: This is the view name that has to be displayed when the URL pattern hits the target.
- Control Aggregation: This refers to the grouping of any UI5 control that this view will be displayed in, such as the App Control’s pages grouping.
- Control ID: The UI5 control’s id, into which this view will be rendered in an aggregate.
- View Type: Javascript, HTML, JSON, and XML. Specifically, what kind of view is intended to be rendered?
Now let’s discuss the next target i.e. MasterView. You can see what settings are made at the router for MasterView in the screenshot below.

Four properties are defined here as well for configuring the target. The view is called MasterView, and the aggregate of SplitApp Control with the id splitappid is called MasterPages. The view is of the XML type.
Moreover, let’s examine DetailView’s settings.

The target of DetailView is configured similarly to that of MasterView. The aggregation of SplitApp Control with the id splitappid is called DetailPages, and the view is named DetailView. DetailView is a kind of XML.
Once the router is configured using the Descriptor editor as demonstrated above, we can see the identical configuration in the Code editor.If you are comfortable with a code editor, you may also carry out the configuration straight in there.
"routing": {
"config": {
"routerClass": "sap.m.routing.Router",
"viewType": "XML",
"async": true,
"viewPath": "org.unity.DemoMasterDetail.view",
"controlId": "app",
"clearControlAggregation": false,
"bypassed": {
"target": []
}
},
"routes": [{
"name": "MasterView",
"pattern": "",
"titleTarget": "",
"greedy": false,
"target": ["MasterView"]
}, {
"name": "SplitAppView",
"pattern": "",
"titleTarget": "",
"greedy": false,
"target": ["SplitAppView"]
}, {
"name": "DetailView",
"pattern": "product/{path}",
"titleTarget": "",
"greedy": false,
"target": ["DetailView"]
}],
"targets": {
"SplitAppView": {
"viewType": "XML",
"viewName": "SplitAppView",
"controlAggregation": "pages"
},
"MasterView": {
"viewType": "XML",
"viewName": "MasterView",
"controlAggregation": "masterPages",
"controlId": "splitappid"
},
"DetailView": {
"viewType": "XML",
"viewName": "DetailView",
"controlAggregation": "detailPages",
"controlId": "splitappid"
}
}
}
Coding SplitAppView’s XML portion
All that the SplitAppView will do is house SplitApp control. It won’t have any additional user interface controls that may be utilized to show business data. This is SplitAppView’s xml code.
<mvc:View xmlns:core="sap.ui.core" xmlns:mvc="sap.ui.core.mvc" xmlns="sap.m"
controllerName="org.unity.DemoMasterDetail.controller.SplitAppView" xmlns:html="http://www.w3.org/1999/xhtml">
<App id="app">
<pages>
<Page title="Online Store">
<content>
<SplitApp id="splitappid" masterButtonText="Master" masterNavigate="" afterMasterNavigate="" masterButton="" detailNavigate="">
<masterPages></masterPages>
<detailPages></detailPages>
</SplitApp>
</content>
</Page>
</pages>
</App>
</mvc:View>

The SplitApp controller won’t require any coding because it’s not necessary.
XML code for MasterView
A List Control will be present in the Master View to show the products’ list.
<mvc:View xmlns:core="sap.ui.core" xmlns:mvc="sap.ui.core.mvc" xmlns="sap.m"
controllerName="org.unity.DemoMasterDetail.controller.MasterView" xmlns:html="http://www.w3.org/1999/xhtml">
<App>
<pages>
<Page id="masterpageid" title="Products" titleLevel="Auto" showNavButton="true" showHeader="true" showSubHeader="true" navButtonText=""
navButtonTooltip="" enableScrolling="true" icon="" navButtonTap="" navButtonPress="">
<content>
<List id="productlist" items="{/Products}" headerText="Products" headerDesign="Standard" footerText="End of the List"
noDataText="No Employees" showNoData="true">
<items>
<ObjectListItem type="Navigation" title="{ProductName}" number="{UnitPrice}" numberUnit="INR" intro="{ProductID}" press="onSelectionChange">
<attributes>
<ObjectAttribute title="" text="{QuantityPerUnit}"></ObjectAttribute>
</attributes>
<firstStatus>
<ObjectStatus title="{UnitsInStock}" text="{designation}"></ObjectStatus>
</firstStatus>
<secondStatus>
<ObjectStatus title="{Discontinued}"></ObjectStatus>
</secondStatus>
</ObjectListItem>
</items>
<headerToolbar>
<Toolbar>
<content>
<Title text="Products" title="Search"></Title>
<ToolbarSpacer/>
<SearchField search="onSearch" width="50%"></SearchField>
</content>
</Toolbar>
</headerToolbar>
</List>
</content>
</Page>
</pages>
</App>
</mvc:View>


MasterView’s Controller code
onInit: function () {
// access OData model declared in manifest.json file
var oModel = this.getOwnerComponent().getModel("myModel");
//set the model on view to be used by the UI controls
this.getView().setModel(oModel);
},
onSelectionChange: function (oEvent) {
// get the source control which triggered this event
var oItem = oEvent.getSource();
// get the binding context of the control
var oCtx = oItem.getBindingContext();
// get the binding path and truncate the first '/'
//so that it does not cause problem when appending the path as navigation //pattern in the url
var sPath = oCtx.getPath().substr(1);
// get the instance of the router and navigate to
//Detail View.
var oRouter = this.getOwnerComponent().getRouter();
oRouter.navTo("DetailView",{path:sPath});
},
It is explained in the comments section of the code above.
The XML Code for DetailView
<mvc:View xmlns:core="sap.ui.core" xmlns:mvc="sap.ui.core.mvc" xmlns="sap.m"
controllerName="org.unity.DemoMasterDetail.controller.DetailView" xmlns:html="http://www.w3.org/1999/xhtml">
<App>
<pages>
<Page id="detailpageid" title="Product Details" titleLevel="Auto" showNavButton="true" showHeader="true" showSubHeader="true"
navButtonText="" navButtonTooltip="" enableScrolling="true" icon="" navButtonTap="" navButtonPress="">
<content>
<ObjectHeader id="objectheaderid" title="{myModel>ProductName}" number="{myModel>UnitPrice}" numberUnit="{myModel>CurrencyCode}">
<statuses>
<ObjectStatus text="Some Damaged" state="Error"/>
<ObjectStatus text="In Stock" state="Success"/>
</statuses>
<attributes>
<ObjectAttribute text="{myModel>SupplierID}"/>
<ObjectAttribute text="{myModel>CategoryID}"/>
<ObjectAttribute text="{myModel>QuantityPerUnit}"/>
<ObjectAttribute text="www.sapyard.com" active="true"/>
</attributes>
</ObjectHeader>
</content>
</Page>
</pages>
</App>
</mvc:View>

A feature of the detail page’s XML view is an ObjectHeader Control that shows data from the Master List’s Selected Item.
The Controller Code for Detail View
onInit: function () {
// get the handle of router
var oRouter = sap.ui.core.UIComponent.getRouterFor(this);
// attach an event to the router which will be fired
//whenever DetailView route is found in the URL Pattern
oRouter.getRoute("DetailView").attachPatternMatched(this._onObjectMatched, this);
},
_onObjectMatched:function(oEvent){
// read the path variable which is passed as part of
// the URL pattern (defined in router configuration)
var sPath = oEvent.getParameter("arguments").path;
// use the path to perform Element binding on the
// ObjectHeader UI control in this view
this.getView().byId("objectheaderid").bindElement({path:"/".concat(sPath), model:"myModel"});
}
The code displayed above includes the necessary comments. Please read carefully to determine the purpose of each sentence.
Run the Program
Select the “run” button located in the Web IDE toolbar. The program will launch in a new browser window and appear as the one below.

Verify the website:
You can notice that the URL does not have a hash pattern (#) at the end by scrolling to the very end. Consequently, the targets that have been set up for a blank pattern are presented. These are the SplitAppView and the MasterView in our instance. When you click on an item in the MasterView, the information below will show.

I made a click on product ID 2.
Check the website again:
Observe how the router entered the picture and added a hash(#) pattern to the URL. There is a Target that this hash pattern resolves to. It will resolve to the DetailView in our instance. Observe that the Pattern is receiving a dynamic parameter called “path” as well. The DetailView uses this dynamic parameter to execute element binding and show the information of the currently clicked product.
Request!
In this guide, I have attempted to provide every detail. However, if you are unfamiliar with UI5 programming, you may still have some inquiries. Feel free to ask them in the comments section below. I respond to all of the feedback.
you may be interested in this blog here:-
How can I see the values of variables in OpenSolver as they change?
Navigating SAP’s E070 Table in SAP: The Heartbeat of Transport Management