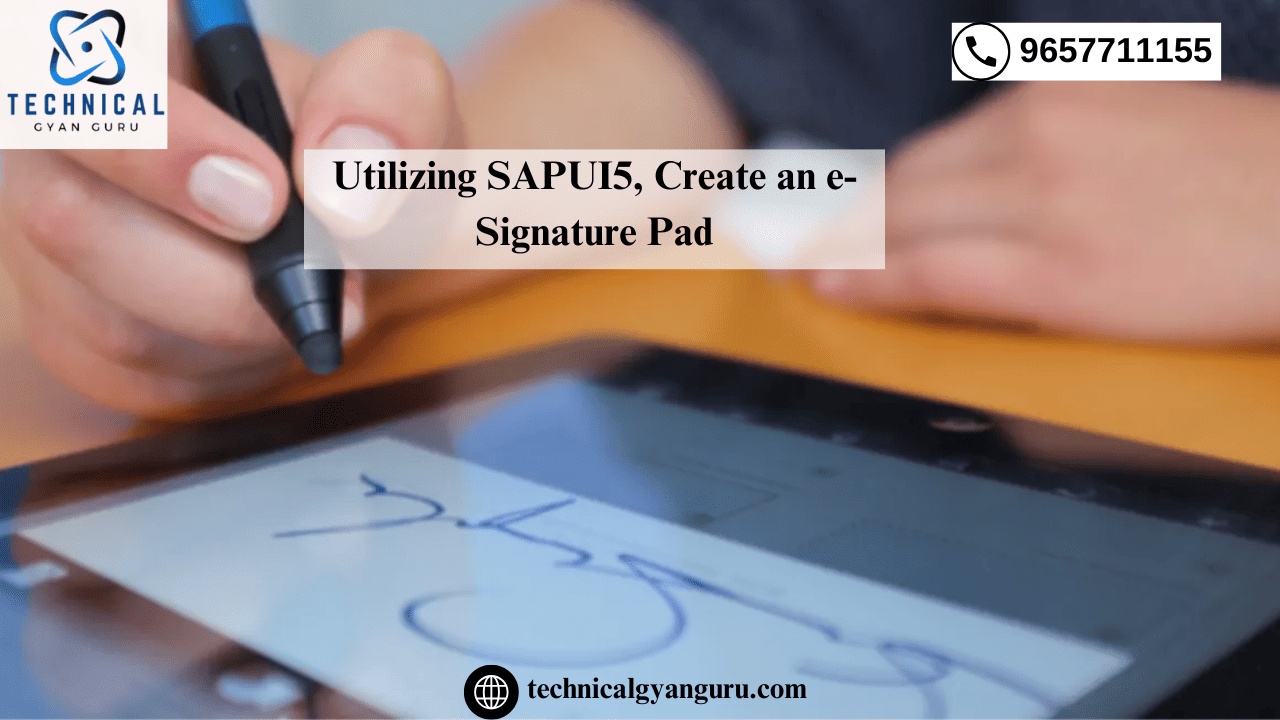
Today, we will learn how to develop an e-signature pad in order to capture customer signatures in a SAPUI5 app, just as promised. To gather ideas, you don’t have to attend SAP Tech Ed in Las Vegas, Barcelona. The spark can come from anything. The courier who delivered some bank documents to my house and collected my e-signature on his portable device served as my inspiration for the e-signature pad. The same, I believed, could be done with SAPUI5. Why not give it a shot?
There are several other blogs where they have used <canvas> tag to achieve this functionality. However, users complaint about the Touch sensitivity not providing the best user experience when ran in Web IDE or actual phone/tablet devices. Interestingly our design works seamlessly in SAP Web IDE and also in actual handheld devices. What different did we do? No rocket science. We have used <svg> tag instead of <canvas> html tag. You need to try it to figure it out
Let’s check how we achieved it.
The e-signature pad does not currently have any standard SAPUI5 control provided. With any luck, SAP is paying attention and will eventually develop a standard one. For the time being, we can design our own Custom Control. I have already published two articles in which we used the
SVG: What is it?
- The acronym for Scalable Vector Graphics is SVG.
- For the Web, vector-based graphics are defined using SVG.
- SVG uses the XML format to define its graphics.
- SVG files support animation for each and every element and property.
- The W3C recommends SVG.
- SVG is compatible with DOM and XSL, among other W3C standards.
Were the words “bouncers” above? unable to comprehend anything at all? Not an issue. When the opposition is in full swing, even the greatest players duck. It is known as honoring the deserving.When I heard these sentences for the first time, it also happened to me.
Assuming we wish to sketch a few lines and a single 300 by 100 rectangle, we can create the HTML code that follows.

Here is the output.

Nice. We can move on with this fundamental information, I believe. The same idea will be applied. When the user puts the mouse cursor over the signature pad, which is the designated area, we’re going to create some curved lines. Similar actions must be performed when the user moves his finger or digital pen over the touch screen of a mobile device because there is no mouse on them.
Utilizing SVG Concepts, Create Custom Controls
sap.ui.core.Control.extend(“customControl.SignaturePad”, { metadata: { properties: { width: {type: ‘int’, defaultValue: screen.availWidth.9}, height: {type: ‘int’, defaultValue: screen.availHeight.58}, bgcolor: {type: ‘string’, defaultValue: ‘#ffffff’}, lineColor: {type: ‘string’, defaultValue: ‘#000000’}, penColor: {type: ‘string’, defaultValue: ‘#000000’}, signature: ‘string’ } }, renderer: function(oRm, oControl) { var bgColor = oControl.getBgcolor(); var lineColor = oControl.getLineColor(); var pen = oControl.getPenColor(); var id = oControl.getId(); var w = oControl.getWidth(); var h = oControl.getHeight(); oRm.write("<div"); oRm.writeControlData(oControl); oRm.write(">"); oRm.write('<svg xmlns="http://www.w3.org/2000/svg" width="' + w + '" height="' + h + '" viewBox="0 0 ' + w + ' ' + h + '">'); oRm.write('<rect id="' + id + '_r" width="' + w + '" height="' + h + '" fill="' + bgColor + '"/>'); var hh = h - 15; oRm.write('<line x1="0" y1="' + hh + '" x2="' + w + '" y2="' + hh + '" stroke="' + lineColor + '" stroke-width="1" stroke-dasharray="3" ' + 'shape-rendering="crispEdges" pointer-events="none"/>'); oRm.write('<path id="' + id + '_p" stroke="' + pen + '" stroke-width="0.5" ' + 'fill="none" pointer-events="none"/>'); oRm.write('</svg>'); oRm.write("</div>"); } |
A few more features, such as the ability to draw lines when a user holds the mouse down button and advances the pointer on a desktop device, should be added. The same applies to mobile devices with touch capabilities; we must manage additional event delegates. The following event delegates need to be added to the Custom Control javascript file.
Event Delegates
/* ‘_r’ is the id of the <rect> html tag which is actually a rectangular panel where the user will put his signature */ var r = document.getElementById(this.getId() + ‘_r’); r.addEventListener(‘mousedown’, down, false); r.addEventListener(‘mousemove’, move, false); r.addEventListener(‘mouseup’, up, false); r.addEventListener(‘touchstart’, down, false); r.addEventListener(‘touchmove’, move, false); r.addEventListener(‘touchend’, up, false); r.addEventListener(‘mouseout’, up, false); |
onAfterRendering Hook Method
onAfterRendering: function() { var that = this; this.signaturePath =”; isDown = false; var elm = this.$()[0]; var r = document.getElementById(this.getId() + ‘_r’); var p = document.getElementById(this.getId() + ‘_p’); var $this = this.$(); var position = $this.position(); $this.css(‘cursor’, ‘pointer’); function isTouchEvent(e) { return e.type.match(/^touch/); } function getCoords(e) { if (isTouchEvent(e)) { return (e.targetTouches[0].clientX - position.left) + ',' + (e.targetTouches[0].clientY - position.top); } return (e.clientX - position.left) + ',' + (e.clientY - position.top); } function down(e) { that.signaturePath += 'M' + getCoords(e) + ' '; p.setAttribute('d', that.signaturePath); isDown = true; if (isTouchEvent(e)) e.preventDefault(); } function move(e) { if (isDown) { that.signaturePath += 'L' + getCoords(e) + ' '; p.setAttribute('d', that.signaturePath); } if (isTouchEvent(e)) e.preventDefault(); } function up(e) { isDown = false; if (isTouchEvent(e)) e.preventDefault(); } r.addEventListener('mousedown', down, false); r.addEventListener('mousemove', move, false); r.addEventListener('mouseup', up, false); r.addEventListener('touchstart', down, false); r.addEventListener('touchmove', move, false); r.addEventListener('touchend', up, false); r.addEventListener('mouseout', up, false); if (this.getSignature()) { console.log('asdasda'); this.signaturePath = this.getSignature(); var p = document.getElementById(this.getId() + '_p'); if (p) { p.setAttribute('d', this.signaturePath); } } this.setSignature = function(s) { this.setProperty('signature', s); this.invalidate(); } } |
Additionally, logic should be written to remove the content of the signature panel when the user clicks the “Clear” button.
Clear Signature:
clear: function() { this.signaturePath = ”; var p = document.getElementById(this.getId() + ‘_p’); p.setAttribute(‘d’, ”); } |
The id of the html tag is ‘_p’.
Our Custom Control for the Signature Pad is now available. They are now usable in the View. What you will write in the XML view looks like this.
XML View
<core:View xmlns:core=”sap.ui.core” xmlns:mvc=”sap.ui.core.mvc” xmlns=”sap.m” xmlns:control=”customControl”> <Page> <content> <control: SignaturePad> </control:SignaturePad> <Label text=”Please sign above” design=”bold” /> <Button text=”Clear” press=”clear” /> </content> </Page> </core:View> |
Let’s Test it.

you may be interested in this blog here:-
Just a key and two clicks for ALV consistency check
Making Your First Salesforce App exchange: A Complete Strategy